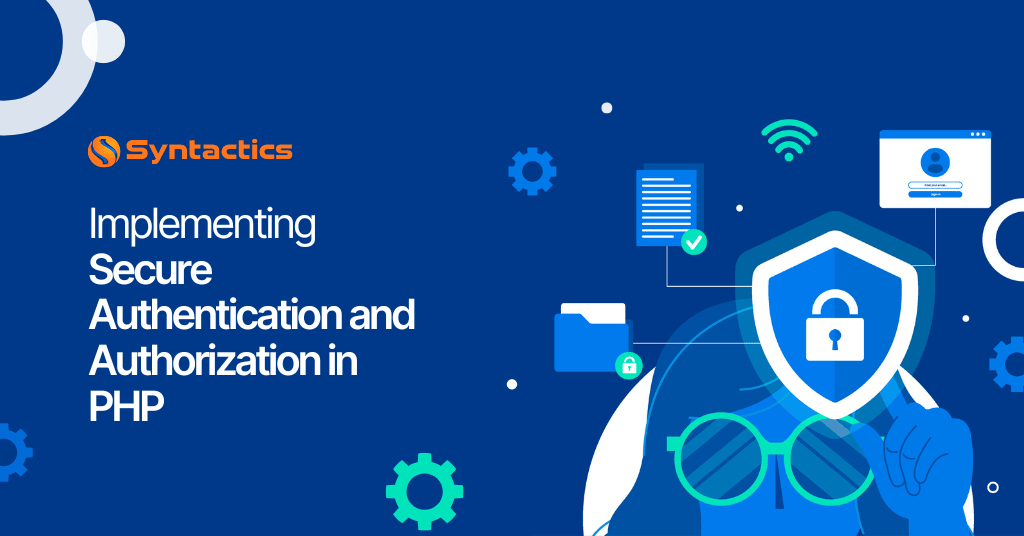
Implementing Secure Authentication and Authorization in PHP
Web applications often handle sensitive user data. Fortunately, secure authentication and authorization mechanisms protect sensitive data and maintain the integrity of applications. An experienced PHP developer is skilled at building secure web apps that protect information from unauthorized access, thus maintaining the app’s integrity.
Source: TechJury.
As a popular server-side scripting language, W3Techs revealed that PHP is utilized by as many as 75.1% of sites with known server-side programming languages.
Fortunately, PHP provides web development experts with enhanced flexibility. It offers various tools and libraries for implementing secure authentication and authorization to protect applications against unauthorized access and potential attacks.
A professional web application development company follows best practices and techniques for building secure PHP apps. These include secure coding practices, data validation, and protection against common security threats.
The Fundamentals of Authentication and Authorization
Before diving into the implementation processes, it’s crucial to understand the two core concepts of secure app development:
Through authentication, a system confirms a user’s identity, often using credentials like usernames, passwords, or biometric data. Authorization determines the actions an authenticated user is allowed to perform on the system or application, such as accessing specific resources.
Typically, authentication and authorization on a PHP app proceeds as follows:
- A user logs into the application, submitting login credentials via a form.
- The server authenticates the credentials against a secure database.
- Upon authentication, the server authorizes the user’s actions based on their role or permissions.
These mechanisms work together to safeguard web applications from vulnerabilities like broken authentication and session hijacking.
Implementing Secure Authentication in PHP
A PHP web application’s authentication mechanism ensures that only verified users can access it. Here’s how developers implement secure authentication:
1. Using Secure Password Management Techniques
Storing passwords in plaintext poses significant security risks. One way web app developers avoid this is through hashing and salting, which are critical approaches for securely storing passwords.
Learn about the PHP coding standards for WordPress here:
Hashing converts passwords into a string of characters, rendering them unreadable. In particular, developers secure password storage and management by utilizing the password_hash() and password_verify() functions.
On the other hand, salting adds a unique random string to each password before the hashing process. This helps defend against pre-computed attacks such as rainbow tables. As a result, even if two users have the same password, their stored hashes will differ because of the unique salts.
Another way to ensure robust web application security is by enforcing strong password policies. Apps can be configured to require passwords that are complex, have a set length (ex. 8-12 characters), and will expire after a certain amount of time.
2. Enhancing Authentication with Multi-Factor Authentication (MFA)
Multi-Factor Authentication requires additional verification steps, significantly reducing the risk of unauthorized access. As such, compromising one factor (like a password) alone is insufficient to gain access to the app.
Common verification methods include biometric checks, OTPs (One-Time Passwords), and TOTP (Time-based One-Time Passwords).
Web developers use various tools to securely store and manage MFA data. For instance, they may implement SMS- or email-based OTPs via third-party services such as SendGrid or Twilio. As for TOTP, a developer can utilize PHP libraries or external ones like Google Authenticator.
3. Implementing Secure Session Management
Session hijacking is a common attack vector. Development experts configure PHP app sessions to enhance web security by using secure cookies and HTTP-only flags.
For the former, web app developers can ensure that cookies are only sent via HTTPS by setting the ‘Secure’ flag on them. As for the latter, the ‘HttpOnly’ flag prevents client-side scripts from accessing session cookies.
Developers regenerate session IDs after successful user logins and at regular intervals throughout the session. This mitigates risks associated with session fixation attacks. Additionally, they can store session data in secure locations to protect from hijacking attacks and unauthorized access.
Learn about debugging and problem-solving strategies for PHP development:
4. Leveraging Modern Authentication Protocols (e.g., OAuth2, JWT)
It’s vital to secure applications by leveraging modern authentication protocols such as OAuth2 and JSON Web Tokens (JWT). The two are token-based protocols that enable secure and stateless authentication, especially for APIs.
Token-based authentication allows stateless sessions, and user credentials aren’t stored on the server.
There are two distinct approaches in managing user interactions in web apps: stateful and stateless sessions. Each has its pros and cons in terms of scalability, fault tolerance, and resource use.
Firstly, stateful sessions, which store user data on the server, enable seamless user interactions. However, it requires more resources and complex scaling.
Stateless sessions store data client-side and treat each user request independently, which improves scalability and resource efficiency. Though it is a preferable option, it requires all information to be sent with every request.
JWTs contain claims about the user and are signed to prevent tampering. This makes them a popular choice for stateless applications. Libraries such as firebase/php-jwt simplify token generation and validation.
Meanwhile, PHP League’s OAuth2 Client library provides a framework for authorization, allowing third-party apps limited access without sharing credentials. In particular, it’s best for web apps that require third-party authentication from providers like Google and Facebook.
Furthermore, web application development experts take care to adhere to the following best practices for securely handling and storing tokens:
- Using HTTPS to transmit to prevent interception or tampering.
- Securely storing tokens on the client-side, whether in memory or secure storage, rather than on local storage or cookies.
- Ensuring that tokens are short-lived by setting expiration times, and limiting their validity period.
- Revoking tokens, such as if a user logs out or if there’s a security breach.
- Validating tokens on each request before granting access.
Implementing Robust Authorization in PHP
Secure authorization on PHP applications specifies what users can do. Here’s how a seasoned developer implements authorization on web apps:
1. Role-Based Access Control (RBAC)
Role-Based Access Control is a crucial mechanism for managing secure authentication and authorization in PHP applications. It organizes access rights based on user roles, ensuring that users can access resources appropriate to their responsibilities.
Professional web app developers define roles and assign permissions based on predefined levels. Then, they store these roles and permissions in a database for scalability.
Developers even utilize several libraries to simplify RBAC implementation and efficiently manage roles and permissions. Examples include PHP-RBAC (for hierarchical role management) and Spatie Laravel Permission (which is ideal for Laravel projects).
See what tools seasoned developers use for Laravel development here:
Lastly, web app developers ensure security by regularly reviewing and updating access controls as roles evolve. Maintaining an effective RBAC system requires several measures, such as:
- Periodically auditing access controls to ensure that they align with current job responsibilities.
- Updating roles and permissions as the application evolves or business needs change.
- Keeping logs of role assignments and permission changes
- Implementing a feedback mechanism to encourage users to report any issues with their access rights or request changes based on responsibilities.
- Conducting thorough testing before implementing significant changes to roles or permissions to prevent unintended access issues.
2. Attribute-Based Access Control (ABAC)
Compared to RBAC, which relies on user roles, Attribute-Based Access Control relies on attributes to enforce access controls. This flexible authorization model enables fine-grained, context-aware access control for complex scenarios.
Examples of user attributes include identity, department or location, resource type, actions, etc. In addition to attributes, ABAC examines resource properties and contextual or environmental factors to dynamically grant or deny access.
Overall, ABAC’s dynamic and adaptable approach makes it ideal for modern, secure PHP applications.
3. Implementing Access Control Checks Throughout the Application
It’s vital to implement access control checks throughout the web app to ensure robust security. Seasoned web developers consistently perform authorization checks across all endpoints and application layers.
They also follow other strategies for enforcing authorization on PHP web applications, such as implementing centralized access control and adopting a “deny all” approach.
Furthermore, validating permissions for sensitive actions prevents privilege escalation.
Lastly, experienced developers regularly audit and test access controls to ensure they function as intended.
Mitigating Common Security Threats in Authentication and Authorization
Developers utilize a variety of strategies to mitigate common web app security threats. These include:
1. Protecting Against Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery attacks trick users into performing unintended actions on a web application.
To safeguard apps against these attacks, it’s critical to implement anti-CSRF tokens to ensure that requests come from authenticated users. Web app developers generate and verify CSRF tokens during form submissions for sensitive actions.
Experienced web app development specialists often rely on PHP frameworks that simplify CSRF protection, like Laravel, Symfony, and CodeIgniter. In addition, they adhere to best practices such as limiting token lifespans and testing for CSRF vulnerabilities.
Source: Statista.
2. Preventing SQL Injection and Code Injection
Structured Query Language and code injection attacks exploit vulnerabilities in handling user input. Effective validation, sanitization, and secure query methods are essential for protecting PHP web applications from such attacks.
Input validation ensures user inputs match expected formats, like using filter_var() to validate integers. Meanwhile, sanitization removes potentially harmful characters to prevent malicious input.
To further reduce risks, limit input lengths and avoid directly concatenating user input into SQL queries. Instead, prioritize secure methods.
Developers use prepared statements and parameterized queries with PHP Data Objects (PDOs) for database interactions. PDOs separate SQL logic from user data, reducing injection risks.
3. Guarding Against Cross-Site Scripting (XSS)
Cross-Site Scripting attacks exploit vulnerabilities to execute malicious scripts in users’ browsers. To mitigate these attacks, it’s critical to sanitize output and securely handle user input.
Developers utilize the htmlspecialchars() function to sanitize user inputs and apply them consistently, especially in templates and views. This PHP function converts special characters (e.g., <, >, &) into harmless HTML entities.
As such, user input is treated as plain text rather than executable code.
It’s also crucial to consider contextual encoding for specific scenarios like URLs or JavaScript.
To secure input handling and prevent XSS attacks, expert developers follow key practices such as input validation, sanitization, limiting input types, securing URL handling, and implementing a Content Security Policy (CSP).
Source: Expert Insights.
Configuring Secure PHP Settings for Authentication and Authorization
There are two approaches to configuring authentication and authorization settings:
1. Securing PHP Configuration Files and Sensitive Information
Properly securing PHP configuration files and sensitive data is critical to protecting your application from unauthorized access and data breaches.
Web app developers store sensitive information like database credentials or API keys in environment variables or .env files instead of configuration files.
Additionally, they avoid hardcoding sensitive data within the web app’s codebase. Instead, credentials are stored in secure, restricted configuration files and regularly audited to remove outdated information.
Another best practice for storing sensitive credentials in PHP applications is using environment variables. This approach ensures that sensitive information stays out of the source code.
To further protect configuration files from unauthorized access, developers can restrict permissions in .htaccess:.
Moreover, experienced developers block direct browser access by storing files outside the web root. For instance, if the root is /var/www/html, they can store configuration files in /var/www/config.
2. Setting PHP Configuration Directives for Security
Properly configuring PHP settings is a crucial step in securing web applications. Experienced developers disable dangerous functions and configure error handling for production.
Some PHP functions, such as those that allow system command execution or file manipulation, can be exploited by malicious actors. By disabling these functions through the disable_functions directive, developers can prevent command injection and other exploits.
Strict error reporting can help avoid exposing sensitive information. Experienced developers can enable error logging to a secure file.
Continuous Monitoring and Auditing for Security
Continuous monitoring and auditing are essential for secure authentication and authorization in PHP applications. Through comprehensive logging, the app records login or authentication attempts and authorization activities for auditing purposes. It captures details such as:
- Successful logins;
- Failed attempts;
- Timestamps, and
- IP addresses.
Meanwhile, monitoring tools detect any suspicious login patterns and breaches and alert administrators. Other best practices include regular penetration testing, scheduled audits, and code reviews.
Conclusion
Secure authentication and authorization are crucial for safeguarding user data and maintaining the integrity of PHP web applications. By embracing a combination of best practices, developers can significantly enhance web app security.
As vulnerabilities continue to evolve, leveraging modern libraries and adhering to key security protocols enable developers to create robust and secure web apps.
Frequently Asked Questions About PHP Web App Security
What is a PHP web app?
A PHP web application is a dynamic system that uses PHP as its server-side scripting language to generate content and interact with databases. Developers can create personalized user experiences on websites.
What is a PHP developer?
A PHP developer specializes in using PHP to build, maintain, and enhance web applications. They are skilled at server-side scripting, database management, and integrating PHP with front-end technologies.
Are PHP developers in demand?
Yes, PHP developers are in demand. Currently, many websites and applications rely on PHP for back-end functionality. The widespread use of PHP in web development leads to ongoing opportunities for skilled developers in the job market.
Comment 0